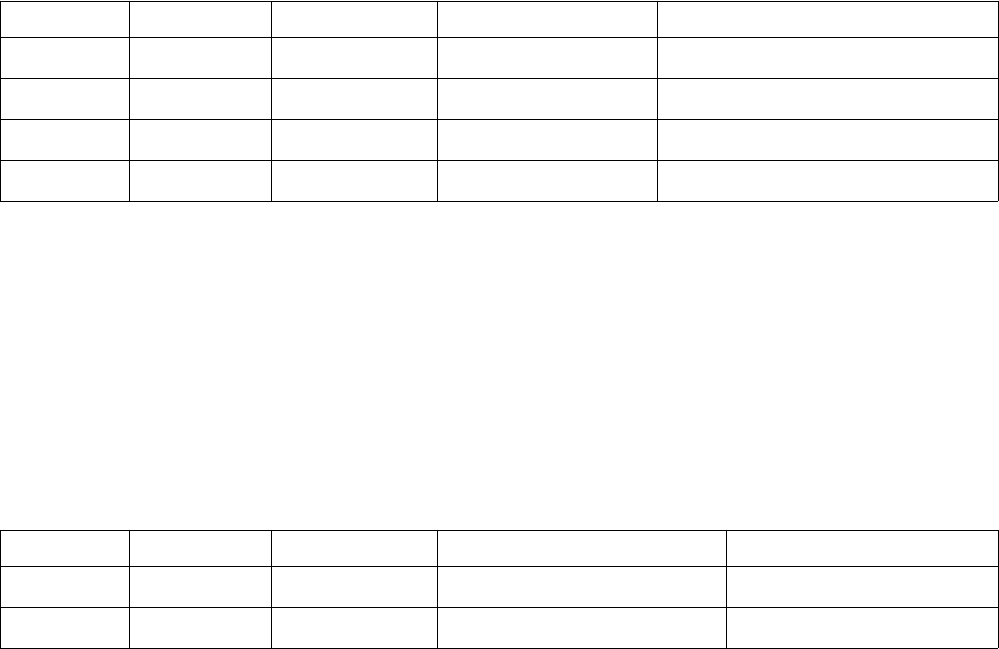
Getting Started With Java – Data Types & Variables
The basic unit of information on a computer is called a bit, which has two states: on or off (1 or 0). Bits
are generally grouped into units of 8, which is called a byte. A single byte can store all of the possible
combinations of the 8 bits, or 2
8
= 256 values.
In Java, as with most programming languages, there are various data types, which are used to
represent and (as variables) store different types of data. Each data type has different demands on
how much space it requires in the computer's memory, and this is measured in bytes.
Integer Data Types
We have already referred to a single byte representing 256 states of the 8 bits. Another way to look at
this is to imagine each of those states representing a number. In the case of a single byte of data,
Java uses the byte integer data type to represent the numbers from -128 to 127.
Type Size (bits) Size (bytes) Range Approximate Range
byte 8 1
short 16 2
int 32 4
long 64 8
The int type is adequate for most tasks and will generally be your default integer data type.
Integer constants must not be written with a decimal point, and they cannot contain any separators
between digits.
For example, each of the following is an illegal integer constant.
(a) 37.0 contains a decimal point
(b) -12 562 contains a blank space between digits
(c) 1,233,985 contains commas between digits
Real Data Types
Type Size (bits) Size (bytes) Precision Approximate Range
float 32 4 at least 6 decimal places
double 64 8 at least 15 decimal places
Due to memory and speed considerations, the float data type was traditionally used more frequently.
With modern computers, however, the double data type does not result in the same performance
issues, and it should be used for most calculations involving real numbers.
Each of the following is a valid floating point constant.
5.23 .3 2818. -0.0002 6.7
Floating point constants can also be written in a form similar to that used in scientific notation.
(a) 5.6e2 represents the value
= 560
(b) 37E-4 represents the value
= 0.0037
(c) -0.667e-2 represents the value
= -0.00667
Page 1 of 5